Container
TL;DR
Lifecycle
docker create
creates a container but does not start it.docker rename
allows the container to be renamed.docker run
creates and starts a container in one operation.docker rm
deletes a container.docker update
updates a container’s resource limits.
Starting and stpping
docker start
starts a container so it is running.docker stop
stops a running container.docker restart
stops and starts a container.docker pause
pauses a running container, “freezing” it in place.docker unpause
will unpause a running container.docker wait
blocks until running container stops.docker kill
sends a SIGKILL to a running container.docker attach
will connect to a running container.
Info
docker ps
shows running containers.docker ps -a
shows running and stopped containers.
docker logs
gets logs from container. (You can use a custom log driver, but logs is only available forjson-file
andjournald
in 1.10).docker inspect
looks at all the info on a container (including IP address).docker events
gets events from container.docker port
shows public facing port of container.docker top
shows running processes in container.docker stats
shows containers’ resource usage statistics.docker stats --all
shows a list of all containers, default shows just running.
docker diff
shows changed files in the container’s FS.
Import/Export
docker cp
copies files or folders between a container and the local filesystem.docker export
turns container filesystem into tarball archive stream to STDOUT.
Executing commands
docker exec
to execute a command in container.
What is Docker container?
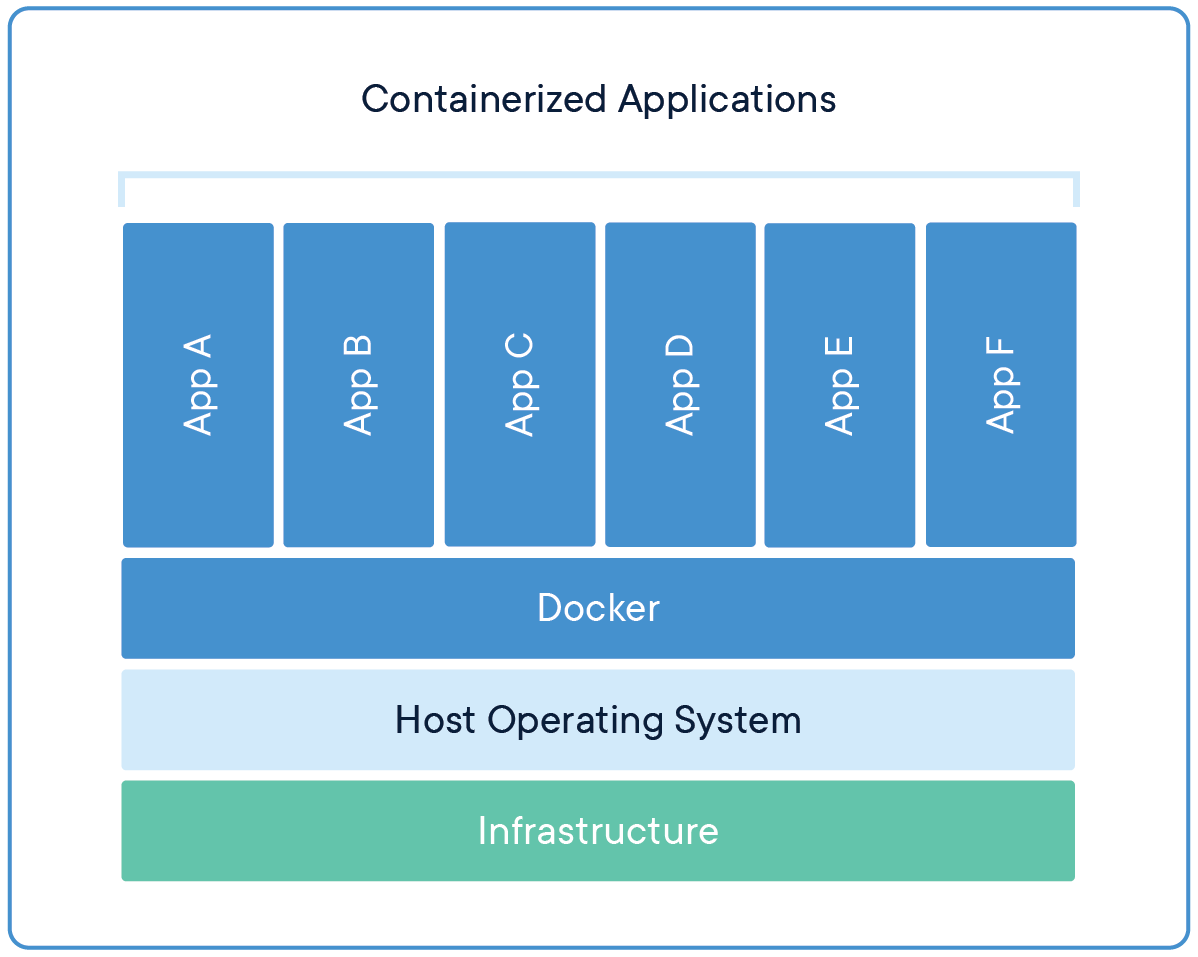
- Containers are an abstraction at the app layer that packages code and dependencies together.
- Multiple containers can run on the same machine and share the OS kernel with other containers, each running as isolated processes in user space.
- Containers take up less space than VMs (container images are typically tens of MBs in size), can handle more applications and require fewer VMs and Operating systems.
Basic Usages
Pulling image
We can use docker pull
to pull images from registry
E.g.: Pull ubuntu
image
docker pull ubuntu
Running a container
Running of containers is managed with the Docker run
command.
For example, we creat a container using an ubuntu:15.10
image and start it:
docker run -it ubuntu:15.10 /bin/bash
-i
: interactive-t
: terminalubuntu:15.10
: ubuntu image (version tag15.10
)/bin/bash
: command behind image
If we want our docker service to run in background, we can use argument -d
. For example:
docker run -itd --name ubuntu-test-1 ubuntu /bin/bash
--name
: assign a nameubuntu-test-1
to this container-d
: If we add this argument, it won’t enter the container by default
Listing containers
Listing running containers:
docker ps
Listing all containers
docker ps -a
Starting a container
docker start <stopped-container-ID>=
Ruuning a command in a running container
docker exec <container-name> <command>
For example, we run a container firstly in background
docker run -itd --name ubuntu-test-1 ubuntu /bin/bash
Check the running container:
docker ps
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
67cbb5de95c2 ubuntu "/bin/bash" 10 minutes ago Up 10 minutes ubuntu-test-1
Now we want to print Hello world
in the terminal of container ubuntu-test-1
:
docker exec -it ubuntu-test-1 echo "Hello World"
Hello World
Stopping a container
docker stop <container-ID>
Removing a container
docker rm -f <container-ID>