JSON
TL;DR
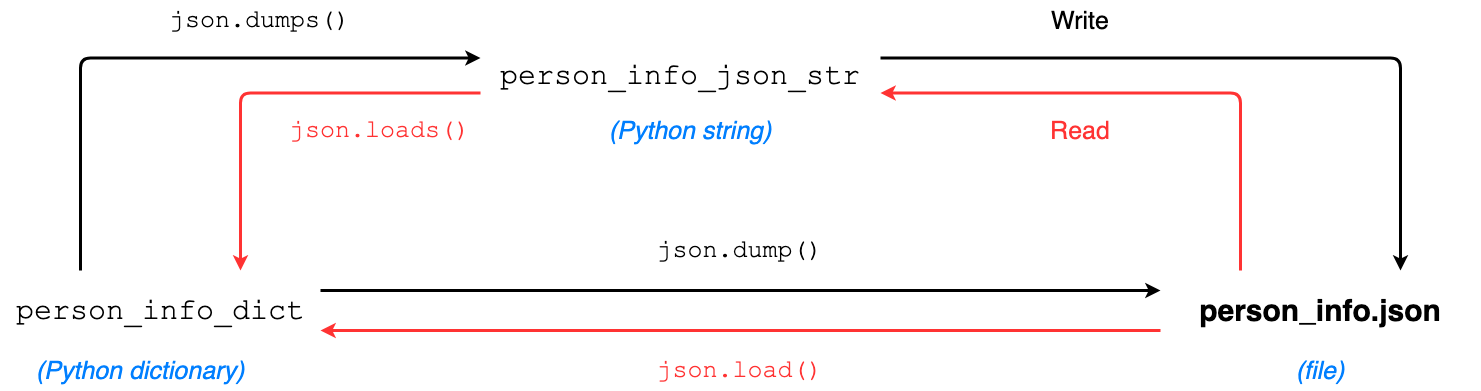
Python and JSON conversion
JSON (JavaScript Object Notation) is a popular data format used for representing structured data. It’s common to transmit and receive data between a server and web application in JSON format.
JSON in Python 3
Python has a built-in package called json
, which can be used to work with JSON data.
import json
JSON to Python
You can parse a JSON string using json.loads()
method. The method returns a dictionary.
For example:
import json
person = '{"name": "Bob", "languages": ["English", "Fench"]}' # JSON string
person_dict = json.loads(person) # Python dictionary
# Output: {'name': 'Bob', 'languages': ['English', 'Fench']}
print(person_dict)
# Output: ['English', 'French']
print(person_dict['languages'])
To read a file containing JSON object, you can use json.load()
method
Suppose, you have a file named person.json
which contains a JSON object.
{
"name": "Bob",
"languages": [
"English",
"Fench"
]
}
Parse this file:
import json
with open('person.json') as f:
data = json.load(f)
# Output: {'name': 'Bob', 'languages': ['English', 'Fench']}
print(data)
Json2Python Conversion table
JSON | Python Equivalent |
---|---|
object | dict |
array | list |
string | str |
number (int) | int |
number (real) | float |
true | True |
false | False |
null | None |
Python to JSON
You can convert a dictionary to JSON string using json.dumps()
method.
import json
person_dict = {
'name': 'Bob',
'age': 12,
'children': None
}
person_json = json.dumps(person_dict)
# Output: {"name": "Bob", "age": 12, "children": null}
print(person_json)
To write JSON to a file in Python, we can use json.dump()
method.
import json
person_dict = {
"name": "Bob",
"languages": [
"English",
"Fench"
],
"married": True,
"age": 32
}
with open('person.json', 'w') as json_file:
json.dump(person_dict, json_file)
When you run the program, the person.txt
file will be created. The file has following text inside it.
{"name": "Bob", "languages": ["English", "Fench"], "married": true, "age": 32}
Python2Json Conversion table
Python | JSON Equivalent |
---|---|
dict | object |
list, tuple | array |
str | string |
int, float, int- & float-derived Enums | number |
True | true |
False | false |
None | null |
Formatting
Indent
Use the indent
parameter to define the numbers of indents. For example:
json.dumps(person_dict, indent=4)
Order the result
Sort keys in ascending order using sort_keys=True
. E.g.:
json.dumps(person_dict, indent = 4, sort_keys=True)